Taking Advantage of the Object Archive Attribute
Introduction
With many web image galleries, when you are looking at a set of images and you click a button to see another set, the new set takes time to arrive and settle. In many of these web sites when you attempt to enlarge an image it would take time before you see the enlarged image. In this article, I show you how to produce the new set of images fast and how to enlarge the images fast, thanks to the Object archive attribute and the use of the image source attribute respectively.

You need basic knowledge in HTML, CSS and JavaScript in order to understand this article. The principles of this article work with Opera 9 and may be the latest versions of new browsers.
The Current Problem and Solution
Images generally take time to download. If the new set of images or the enlarged image has to come from the server, while the user is viewing the gallery, then time would be taken for the images to arrive at the client computer.
Today, with the Object archive attribute, it is possible to have all the images of the gallery at the client browser. When a new image (set) is needed by the user, it is got from the archive attribute at the client, and not from the server. There is no time wasted downloading from the server. This leads to fast operation of the image gallery.
The first set of images may take time to download, and that is the only time the user would perceive. As the user is busy looking at the first set, the rest of the sets (of images) are downloaded. After the first set, if he needs any image, it is got from the archive attribute.
The Object Archive Attribute
HTML has an element called the OBJECT element. This object has an attribute called the archive attribute. The value of this attribute is a space-separated list of URLs. As soon as the web page is loaded, each of these URLs downloads its resource (code, image, video, etc.). The resources arrive at the browser and just stay there until they are needed. These resources are officially there to be used by the Object element. However, the recourses can still be used by other HTML elements.
Regular Expression Technique for Separating the URLs
To conveniently separate the URLs in the string value of the archive attribute, you need Regular Expression Technique. Consider the following statement:
var subject = document.getElementById(ID).archive;
This statement copies the archive attribute string into the variable, subject. The following statement gets all the URLs into the array, arr.
arr = subject.match(/\S+/g);
After the above statement the archive attribute string with its URLs remains as it was; Each element in the array has a URL copied from the archive attribute. The first URL of the archive attribute is the first element of the array; the second URL of the archive attribute is the second element of the array; the third URL is the third element of the array and so on. I will not explain the second statement above any further, if you want to know more about it, read my series titled, JavaScript String Regular Expressions, in this blog. To arrive at the series, type, JavaScript String Regular Expressions, in the Search box of this page and click Search. If you have the Google Search box on the page, use it.
A Project
I use a project for illustration. You can modify the project for your commercial purposes. In this project, there are three sets of images: each set consists of 5 images. This gives a total of 15 images for the gallery. In practice, you can have more than 15 images and more than 5 images per set. However, for our pedagogic purpose here, we have just 15, in sets of 5.
All the images will be displayed on one web page. Only one image can be enlarged at any one time. One set of 5 images is always displayed; each of these 5 images is displayed in a small size, in a row. When you click any of these 5 images, you see the enlarged version in an area above the row.
The first set of images is downloaded with the web page. The next two sets are downloaded by an Object’s archive attribute. For consistency, the first set will also be listed in the archive attribute. Now, when an image source value (URL) occurs more than once in a web page, the web browser does not really download it more than once. The browser simply downloads the image once and caches it. When next the image (resource) is needed again in the page, it gets it from the cache. Some browsers (especially old versions), may not do this, but many browsers in use today do this.
You should see the first set of images when the page opens. The first set of images take the normal time to download, so if the Internet line is slow, you (the user) might notice some time delay as the first set of images arrive. After this you will notice no more delays, because as you are busy looking at the first set and enlarging its images, one-by-one, the other two sets are being downloaded by the archive attribute. They come and they remain in the archive attribute. Whenever they are needed, they will be got from the archive attribute and not from the server. So the download time from the server will be omitted, and the user will notice a fast display of all the images after the first set has arrived.
Enlarging an Image
As said above, each set of images displays five images in a row. When you click one of the images, you see its enlarged form above it. As part of the design, when the first set of images arrives at the browser, you see the first image (left-most in the row) enlarged.
The enlarged image simply has larger dimensions than the images in the row (that are not enlarged). These larger dimensions are given by CSS. When you click any small image, the value (URL) of the source attribute of the larger image is replaced by that of the smaller image. When the replacement takes place, you see the image clicked enlarged (higher up). For any enlarged image, you have the same URL for the image tag of the enlarged image and the image tag of the corresponding small image. It is the source attribute of an image tag that determines what the image tag displays as the image.
Resolution
The enlarged image, in pixels, should not be larger than any of the images at the server. If you do not respect this rule, there will be lost of resolution of one or more of the enlarged image.
Let us go on to implement the project.
Implementing the Project
The code is in 3 parts. The first part is the style sheet in the HTML HEAD element. The next part is the JavaScript. The third part is the content of the BODY element; this content has the HTML elements of interest: the object element, the table elements, the image elements and the button element.
Code for BODY Content
We look at the content of the BODY element first, since the style sheet and the JavaScript depend on it. This is it:
Some text can go here.Some text can go here.
Next Set
The Body tags have been included in the above code. In the start tag of the Body element, you have the attribute/value pair, onload=”fillArray()”. This is the onload event that calls the JavaScript function, fillArray(). This function uses JavaScript regular expression technique to copy the list of URLs of the object’s archive attribute into an array.
Now, into the Body content: The first element you have in the Body content is the Object element. We need this Object element just because of its archive attribute. So the only important thing the Object element has is the archive attribute and the value of this attribute. The Object element as coded does not occupy space in the web page. The value of the archive attribute is a space-separated list of URLs. Because these URLs are of the archive attribute, their resources are downloaded, while the web page user is still beginning to realize what is on the web page. By the time the user needs the images of the URLs, their images would have been downloaded (arrive at the browser).
The next major elements you have are the HTML Table for the enlarged image, the HTML Table for the small images and the button. The Table for the enlarged image has one row and three cells; the cell at the center holds the enlarged image. It is the style sheet that primarily determines the size of an image (both the enlarged and small images).
The second Table has one row and 5 cells; each holds a small image. It is the style sheet that primarily determines the size of an image. After this table, you have an HTML button in the code. It has an onclick event that calls the function, nextSet(). This JavaScript function copies the next set of images whose URLs are now in an array, into the image tags of the second table.
The Style Sheet
This is the simple style sheet:
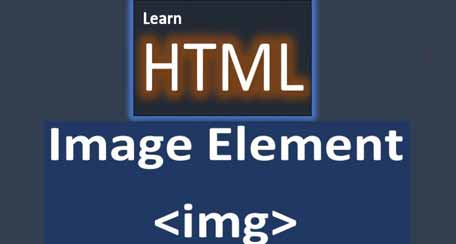
The first statement in the style sheet deals with the small images that have the class, small. The second statement deals with the one enlarged image. This image has the class, large. The declaration block of the first statement has only the width value for the small images. The declaration block for the large image also has only the width value of the large image. Whenever, you give the width value to an HTML element the browser gives the corresponding or appropriate height. Avoid giving height values to HTML elements; this complicates browser presentation with today’s browsers.
The JavaScript
This is the script:
var arr = new Array;
function fillArray()
{
var subject = document.getElementById(‘O1’).archive;
arr = subject.match(/\S+/g);
}
function enlarge(event)
{
document.getElementById(‘I0’).src = event.target.src;
}
var nextIndex = 5;
function nextSet()
{
document.getElementById(‘IS0’).src = arr[nextIndex + 0];
document.getElementById(‘IS1’).src = arr[nextIndex + 1];
document.getElementById(‘IS2’).src = arr[nextIndex + 2];
document.getElementById(‘IS3’).src = arr[nextIndex + 3];
document.getElementById(‘IS4’).src = arr[nextIndex + 4];
//Make enlarged image the first in the set.
document.getElementById(‘I0’).src = arr[nextIndex+0];
nextIndex = nextIndex + 5
if (nextIndex == 15)
{
nextIndex = 0;
document.getElementById(‘B1’) = “First Set”
}
else
document.getElementById(‘B1’) = “Next Set”
}
There are three functions in the JavaScript. The first one is fillArray(), which copies the list of space separated image URLs from the Object’s archive attribute into an array. This function is called, by the BODY onload event. The second one is the enlarge(event) function. This function enlarges a small image when it is clicked. The last function is the nextSet() function. This function replaces the set of small images with the next set. There are two global variables, arr and nextIndex. arr is used by the first and third functions. nextIndex is used only by the third function.
Function Details
The fillArray() function called by the BODY onload event has two statements. The first one copies the value of the Object’s archive attribute as a string into the variable, subject. The second statement uses regular expression technique to separate the URLs from the string and puts them as individual elements in the global array, arr. Note: the fillArray() function does not copy the resources (image) into the array; it copies the texts of the URLs of the archive attribute into the array. These texts are downloaded with the HTML document page first. After the downloading of the HTML document page the downloading of the actual images begin. So, the texts can be copied into the array before the downloading of the images based on the texts (in the archive attribute).
The function enlarge(event) displays the enlarged image for the small image that has been clicked. It simply copies the value of the source attribute of the image clicked, to the value of the source attribute of the image tag in the second cell of the first table. When this copy is made, the image in the second cell takes the dimensions specified by the style sheet. Note the use of the event object in the statement of this function. Old browsers do not support the use of the event object. When an HTML element is clicked, the target attribute of the event object returns a reference to the element that triggered the event.
Now, to the nextSet() function: This function displays the next set of 5 small images. The first set is displayed with the page downloaded. After this, the index for the array that begins the next set of image URLs is 5 (index counting begins from zero). This is why you have the global variable and initialization,
var nextIndex = 5;
When the button is clicked, the first code segment of the nextSet() function copies the next set of the image URLs from the array into the second table image tags. The HTML image tags of the second table are not changed. It is the value of their source attributes that are changed.
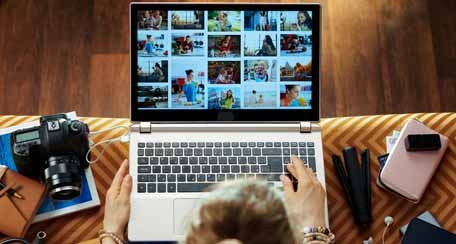
After this code segment, the next statement makes an enlarged copy of the new first image of the second table. After that the nextIndex variable is increased by 5, since we have images in sets of 5. The last code segment tests if nextIndex is 15. If it is 15, then the sets have to be displayed from the beginning. The code inside the “if” and “else” block should be self-explanatory.
The complete code with 15 images can be downloaded from
http://www.cool-mathematics.com/downloads/fastGallery.zip
Conclusion
The principles outlined, work with Opera 9 and maybe other major browsers at their latest versions. I have not been able to test it with these latest browsers (e.g. IE8). You can do the test. So, a secret for fast operation of an image gallery is to take advantage of the functioning of the archive attribute of the HTML Object element.
- 5 Reasons to Try ProHydrolase for Digestive Health - June 20, 2024
- SMS Verification: A Comprehensive Overview - June 10, 2024
- 4 Effective Ways to Manage Erectile Dysfunction - June 3, 2024